ANSIC <<
Previous Next >> 程式解釋
ANSIC_T
1
#include <stdio.h>
int main() {
int x; // 用於存儲總里程的變數,以公里為單位
float y; // 用於存儲總耗油量的變數,以升為單位
// 提示用戶輸入總里程並存入 'x'
printf("Input total distance in km: ");
// 檢查 scanf 的返回值,確保成功讀取一個整數
if (scanf("%d", &x) != 1) {
fprintf(stderr, "Error: Invalid input for total distance.\n");
return 1;
}
// 提示用戶輸入總耗油量並存入 'y'
printf("Input total fuel spent in liters: ");
// 檢查 scanf 的返回值,確保成功讀取一個浮點數
if (scanf("%f", &y) != 1) {
fprintf(stderr, "Error: Invalid input for total fuel spent.\n");
return 1;
}
// 計算並打印平均油耗
printf("Average consumption (km/lt) %.3f ", x / y);
printf("\n");
return 0;
}
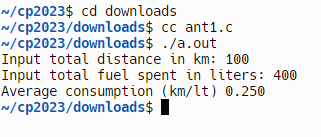
2
#include <stdio.h>
int main() {
float x, y, z, P; // 宣告變數,用於存儲三角形的邊長和周長
// 提示用戶輸入三個邊長並存入 'x', 'y', 和 'z'
printf("\nInput the first number: ");
if (scanf("%f", &x) != 1) {
fprintf(stderr, "Error: Invalid input for the first number.\n");
return 1;
}
printf("\nInput the second number: ");
if (scanf("%f", &y) != 1) {
fprintf(stderr, "Error: Invalid input for the second number.\n");
return 1;
}
printf("\nInput the third number: ");
if (scanf("%f", &z) != 1) {
fprintf(stderr, "Error: Invalid input for the third number.\n");
return 1;
}
if (x < (y + z) && y < (x + z) && z < (y + x)) // 檢查是否可以構成三角形
{
P = x + y + z; // 計算周長
printf("\nPerimeter = %.1f\n", P); // 輸出周長
} else {
printf("Not possible to create a triangle..!"); // 如果無法構成三角形,輸出相應的信息
}
return 0;
}
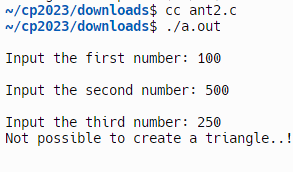
3
#include <stdio.h>
int main() {
int j, numbers[5], total = 0; // 宣告數組用於存儲 5 個數字和變數用於總和
// 提示用戶輸入五個數字並存入數組
for (j = 0; j < 5; j++) {
printf("\nInput the %s number: ", j == 0 ? "first" : (j == 1 ? "second" : (j == 2 ? "third" : (j == 3 ? "fourth" : "fifth"))));
if (scanf("%d", &numbers[j]) != 1) {
fprintf(stderr, "Error: Invalid input for the %s number.\n", j == 0 ? "first" : (j == 1 ? "second" : (j == 2 ? "third" : (j == 3 ? "fourth" : "fifth"))));
return 1;
}
}
for (j = 0; j < 5; j++) {
if ((numbers[j] % 2) != 0) // 檢查數字是否為奇數
{
total += numbers[j]; // 將奇數添加到總和中
}
}
printf("\nSum of all odd values: %d", total); // 輸出所有奇數的總和
return 0;
}
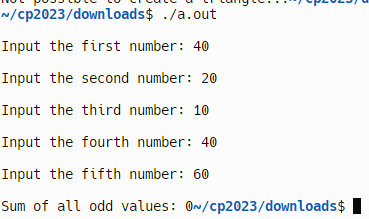
4
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
int divide_two(int dividend_num, int divisor_num) {
int sign = (float)dividend_num / divisor_num > 0 ? 1 : -1;
unsigned int dvd = dividend_num > 0 ? dividend_num : -dividend_num;
unsigned int dvs = divisor_num > 0 ? divisor_num : -divisor_num;
unsigned int bit_num[33];
unsigned int i = 0;
long long d = dvs;
bit_num[i] = d;
while (d <= dvd) {
bit_num[++i] = d = d << 1;
}
i--;
unsigned int result = 0;
while (dvd >= dvs) {
if (dvd >= bit_num[i]) {
dvd -= bit_num[i];
result += (1 << i);
} else {
i--;
}
}
if (result > INT_MAX && sign > 0) {
return INT_MAX;
}
return (int)result * sign;
}
int main(void) {
int dividend_num = 15;
int divisor_num = 3;
printf("Quotient after dividing %d and %d: %d", dividend_num, divisor_num, divide_two(dividend_num, divisor_num));
return 0;
}

5
#include <stdio.h>
// inline function to check if a given integer is even
inline int is_even(int n) {
return n % 2 == 0;
}
int main() {
int num;
// 檢查 scanf 的返回值,確保成功讀取一個整數
if (scanf("%d", &num) != 1) {
fprintf(stderr, "Error: Invalid input. Please enter a valid integer.\n");
return 1;
}
if (is_even(num)) {
printf("%d is even.\n", num);
} else {
printf("%d is odd.\n", num);
}
return 0;
}
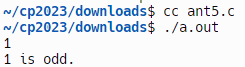
6
#include <stdio.h>
inline float celsius_To_Fahrenheit(float celsius) {
return (celsius * 9 / 5) + 32;
}
inline float fahrenheit_To_Celsius(float fahrenheit) {
return (fahrenheit - 32) * 5 / 9;
}
int main() {
float celsius = 40;
float fahrenheit = 89.60;
printf("%.2f Celsius is equal to %.2f Fahrenheit\n", celsius, celsius_To_Fahrenheit(celsius));
printf("\n%.2f Fahrenheit is equal to %.2f Celsius\n", fahrenheit,
fahrenheit_To_Celsius(fahrenheit));
return 0;
}
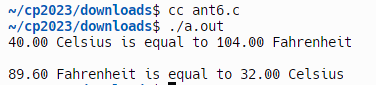
7
#include <stdio.h>
int main() {
int a, b;
// Prompt user for input
printf("Input two pairs values (integer values):\n");
// Read two integer values from user and store them in 'a' and 'b'
if (scanf("%d %d", &a, &b) != 2) {
fprintf(stderr, "Error: Invalid input. Please enter two integer values.\n");
return 1; // Return an error code for invalid input
}
// Check if 'a' is not equal to 'b'
if (a != b) {
// Check if 'b' is greater than 'a'
if (b > a) {
printf("Ascending order\n"); // Print message for ascending order
} else {
printf("Descending order\n"); // Print message for descending order
}
}
return 0; // End of program
}
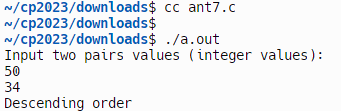
8
#include <stdio.h>
int main(void) {
unsigned char char1, char2, char3, char4, char5, char6, char7, char8;
// Print table header
printf("|---------------------------------------------------------------------------------------------------------|\n");
printf("|extended ASCII table - excluding control characters |\n");
printf("| Ch Dec Hex | Ch Dec Hex | Ch Dec Hex | Ch Dec Hex | Ch Dec Hex | Ch Dec Hex | Ch Dec Hex |\n");
printf("|----------------|----------------|-------------|--------------|--------------|-------------|-------------|\n");
// Loop through characters
for (int i = 0; i < 32; i++) {
// Calculate characters for different ranges
char1 = i;
char2 = i + 32;
char3 = i + 64;
char4 = i + 96;
char5 = i + 128; // extended ASCII characters
char6 = i + 160;
char7 = i + 192;
char8 = i + 224;
// Print characters and their decimal and hexadecimal representations
printf("| %c %3d %#x ", char2, char2, char2);
printf("| %c %3d %#x ", char3, char3, char3);
// Special case for DEL character
if (char4 == 127) {
printf("|%s %3d %#x |", "DEL", char4, char4);
} else {
printf("| %c %3d %#x |", char4, char4, char4);
}
// Print extended ASCII characters for the current system.
printf(" %c %3d %#x | %c %3d %#x | %c %3d %#x | %c %3d %#x |\n",
char5, char5, char5,
char6, char6, char6,
char7, char7, char7,
char8, char8, char8);
}
return 0; // Indicate successful execution of the program
}
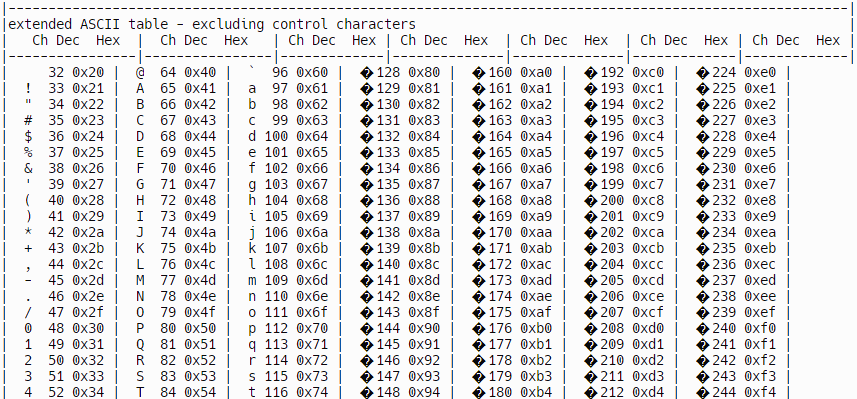
9
#include <stdio.h>
int main() {
int x; // Declare variable x
// Print header for the table
printf("x+1\tx+3\tx+5\tx+7\n\n");
printf("---------------------------\n");
// Loop to generate and print table values
for (x = 1; x <= 15; x += 3)
printf("%d\t%d\t%d\t%d\n", x, (x + 2), (x + 4), (x + 6));
return 0; // Indicate successful program execution
}
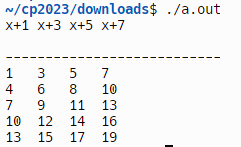
10
#include <stdio.h>
int main() {
// 声明变量
float principal_amt, rate_of_interest, days, interest;
const int yearInDays = 365; // 用于转换利率的常量
// 提示用户输入贷款金额
printf("输入贷款金额 (输入 0 退出): ");
if (scanf("%f", &principal_amt) != 1) {
fprintf(stderr, "Error: Invalid input for loan amount.\n");
return 1;
}
// 主循环用于处理贷款
while ((int)principal_amt != 0)
{
// 提示用户输入利率
printf("输入利率: ");
if (scanf("%f", &rate_of_interest) != 1) {
fprintf(stderr, "Error: Invalid input for interest rate.\n");
return 1;
}
// 提示用户输入贷款期限(天)
printf("输入贷款期限(天): ");
if (scanf("%f", &days) != 1) {
fprintf(stderr, "Error: Invalid input for loan term.\n");
return 1;
}
// 计算利息
interest = (principal_amt * rate_of_interest * days) / yearInDays;
// 显示利息金额
printf("利息金额为 $%.2f\n", interest);
// 提示用户输入下一个贷款金额
printf("\n\n输入贷款金额 (输入 0 退出): ");
if (scanf("%f", &principal_amt) != 1) {
fprintf(stderr, "Error: Invalid input for loan amount.\n");
return 1;
}
}
return 0;
}
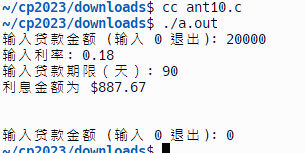
ANSIC <<
Previous Next >> 程式解釋