w11-12 <<
Previous Next >> c_EX
w16
第一題
#include <stdio.h>
#include <gd.h>
// Function to draw the mass-spring-damper system
void draw_system(const char* filename) {
int width = 600;
int height = 300;
int x_margin = 50;
int y_margin = 50;
int mass_radius = 20;
int spring_width = 4;
int damper_width = 4;
gdImagePtr img = gdImageCreate(width, height);
int background_color = gdImageColorAllocate(img, 255, 255, 255);
int mass_color = gdImageColorAllocate(img, 0, 0, 0);
int spring_color = gdImageColorAllocate(img, 0, 0, 0);
int damper_color = gdImageColorAllocate(img, 0, 0, 0);
int wall_color = gdImageColorAllocate(img, 0, 0, 0);
// Draw left wall
gdImageLine(img, x_margin, y_margin, x_margin, height - y_margin, wall_color);
// Draw mass 1
int x1 = x_margin + 2 * mass_radius;
int y1 = height / 2;
gdImageFilledEllipse(img, x1, y1, mass_radius, mass_radius, mass_color);
// Draw spring 1
int spring1_start_x = x_margin;
int spring1_end_x = x1 - mass_radius;
int spring1_y = y1;
gdImageLine(img, spring1_start_x, spring1_y, spring1_end_x, spring1_y, spring_color);
gdImageSetThickness(img, spring_width);
gdImageLine(img, spring1_start_x, spring1_y, spring1_end_x, spring1_y, spring_color);
gdImageSetThickness(img, 1);
// Draw damper 1
int damper1_start_x = x_margin / 2;
int damper1_end_x = x1 - mass_radius;
int damper1_y = y1;
gdImageLine(img, damper1_start_x, damper1_y, damper1_end_x, damper1_y, damper_color);
gdImageSetThickness(img, damper_width);
gdImageLine(img, damper1_start_x, damper1_y, damper1_end_x, damper1_y, damper_color);
gdImageSetThickness(img, 1);
// Draw mass 2
int x2 = width - x_margin - 2 * mass_radius;
int y2 = height / 2;
gdImageFilledEllipse(img, x2, y2, mass_radius, mass_radius, mass_color);
// Draw spring 2
int spring2_start_x = x2 + mass_radius;
int spring2_end_x = width - x_margin;
int spring2_y = y2;
gdImageLine(img, spring2_start_x, spring2_y, spring2_end_x, spring2_y, spring_color);
gdImageSetThickness(img, spring_width);
gdImageLine(img, spring2_start_x, spring2_y, spring2_end_x, spring2_y, spring_color);
gdImageSetThickness(img, 1);
// Draw damper 2
int damper2_start_x = width - x_margin + mass_radius;
int damper2_end_x = x2 + mass_radius;
int damper2_y = y2;
gdImageLine(img, damper2_start_x, damper2_y, damper2_end_x, damper2_y, damper_color);
gdImageSetThickness(img, damper_width);
gdImageLine(img, damper2_start_x, damper2_y, damper2_end_x, damper2_y, damper_color);
gdImageSetThickness(img, 1);
// Draw right wall
gdImageLine(img, width - x_margin, y_margin, width - x_margin, height - y_margin, wall_color);
// Save the image to a file
FILE *output_file = fopen(filename, "wb");
gdImagePng(img, output_file);
fclose(output_file);
// Free the memory used by the image
gdImageDestroy(img);
}
int main() {
draw_system("mass_spring_damper_system.png");
return 0;
}
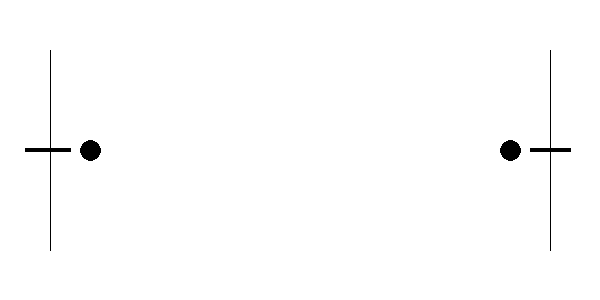
第二題
#include <stdio.h>
// System parameters
#define M1 2.0
#define M2 3.0
#define K1 0.5
#define K2 1.0
#define K3 15.0
#define C1 0.25
#define C2 0.33
#define C3 0.5
// Function to calculate the derivative of the state
void calculate_derivative(double t, double state[4], double derivative[4]) {
derivative[0] = state[2]; // dx1/dt = v1
derivative[1] = state[3]; // dx2/dt = v2
double delta_x = state[0] - state[1];
// dv1/dt
derivative[2] = -(K1 * state[0] + K2 * delta_x) / M1;
// dv2/dt
derivative[3] = -(K3 * state[1] - K2 * delta_x) / M2;
}
// Euler's Method for solving the system
void euler_method(double t_initial, double t_final, double dt, double initial_conditions[4]) {
FILE *output_file;
output_file = fopen("trajectory_data.txt", "w");
double t = t_initial;
double state[4];
for (int i = 0; i < 4; ++i) {
state[i] = initial_conditions[i];
}
while (t <= t_final) {
fprintf(output_file, "%f %f %f %f %f\n", t, state[0], state[1], state[2], state[3]);
double derivative[4];
calculate_derivative(t, state, derivative);
for (int i = 0; i < 4; ++i) {
state[i] += derivative[i] * dt;
}
t += dt;
}
fclose(output_file);
}
int main() {
// Define the initial conditions
double initial_conditions[4] = {1.0, -0.5, 0.0, 0.0}; // x1, x2, v1, v2
// Time parameters
double t_initial = 0.0;
double t_final = 10.0;
double dt = 0.01;
// Solve the system using Euler's Method
euler_method(t_initial, t_final, dt, initial_conditions);
return 0;
}
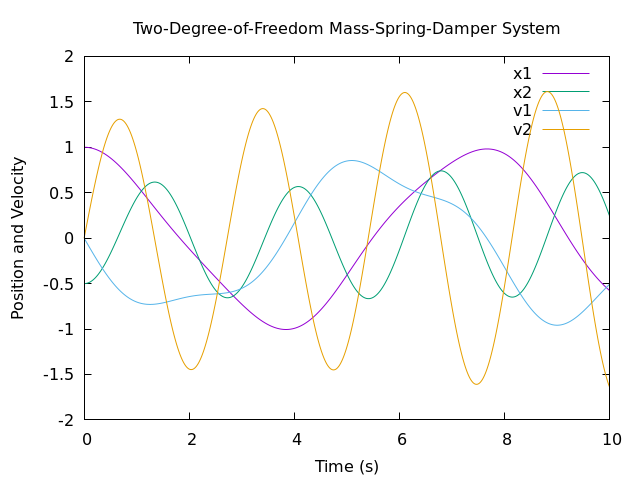
w11-12 <<
Previous Next >> c_EX